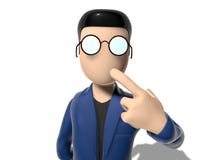
If only I could find an array that could shrink when you remove something. And one that you didn't have to loop through to check each element , but instead you could just ask it if it contains what you are looking for.That would be dreamy. But I know it's just a fantasy...........
WAKE UP AND CHECK THE JAVA LIBRARY.
As if by magic there really is such a thing . It's not a array its an ArrayList , a class in the core Java library (the API). There are hundreds of pre-built classes inside the library , you can use them directly in your code.
They are pre-compiled too !!
FUNCTIONS OF ARRAY LIST
(I) add (Object elem)
Adds the object parameter to the list.
(II) remove (int index)
Removes the object at the index parameter.
(III) remove (Object elem)
Removes the object elem from the list.
(IV) isEmpty ()
Returns 'true' if the list has no elements.
(V) indexOf(Object elem)
Either returns the index of the parameter or -1.
(VI) size()
Returns the number of elements currently in the list.
(VII) get (int index)
Returns the object currently at the index parameter.
ARRAY
LIST
|
ARRAY
|
ArrayList<String> myList=new
ArrayList<String>();
|
String [ ] myList =new String [2];
|
String a = new
String("wohoo");
|
String
a = new String("wohoo");
|
myList.add(a);
|
myList[0] = a;
|
String b = new
String("frog");
|
String b = new String("frog");
|
myList.add(b);
|
myList[1] = b;
|
int theSize = myList.size();
|
int theSize =
myList.length;
|
String o = myList.get(1);
|
String o = myList[1];
|
myList.remove(1);
|
myList[1]
= null;
|
boolean isIn = myList.contains(b);
|
boolean
isIn=false;
for( String item : myList ) {
if ( b.equals(item) ){
isIn = true ;
break ;
} }
|
Notice that we are using the object of type Even though array is an object we can not invoke ArrayList and we are accessing methods any methods on it , although we can access
of the ArrayList using the dot operator. its instance variable , length.
-----------------------------------------------------------------------------------------------------------------------------------
HOW ARRAYLIST IS BETTER THAN ARRAY
-> A array has to know its size at the time it's created. Eg : new String[2]
But for a ArrayList , you can just make an object , there is no need to specify its size . It can easily grow and shrink as the objects are added and removed. Eg : new ArrayList<String>()
-> To insert an object into the array we need to specify the index. Eg : myList[1] = b; And if it exceed the size of the array it creates exception at runtime.
But an arrayList simply requires you to add the object , there is no need to specify the index . Eg : myList.add(b) ; And it will keep growing to make room for more elements in the list.
-> To know whether the element is present in the array we need to write 5-6 lines of code.
Whereas this job could be done easily by using an ArrayList as shown in the above example.
-------------------------------------------------------------------------------------------------------------------------------------
Nice post !!
ReplyDeleteArray List is definitely better than array.
Do we need to include something before using ArrayList in our program??Like we do in C by including header files??
ReplyDeleteYes, we need to add a import --> import java.util.ArrayList
ReplyDelete